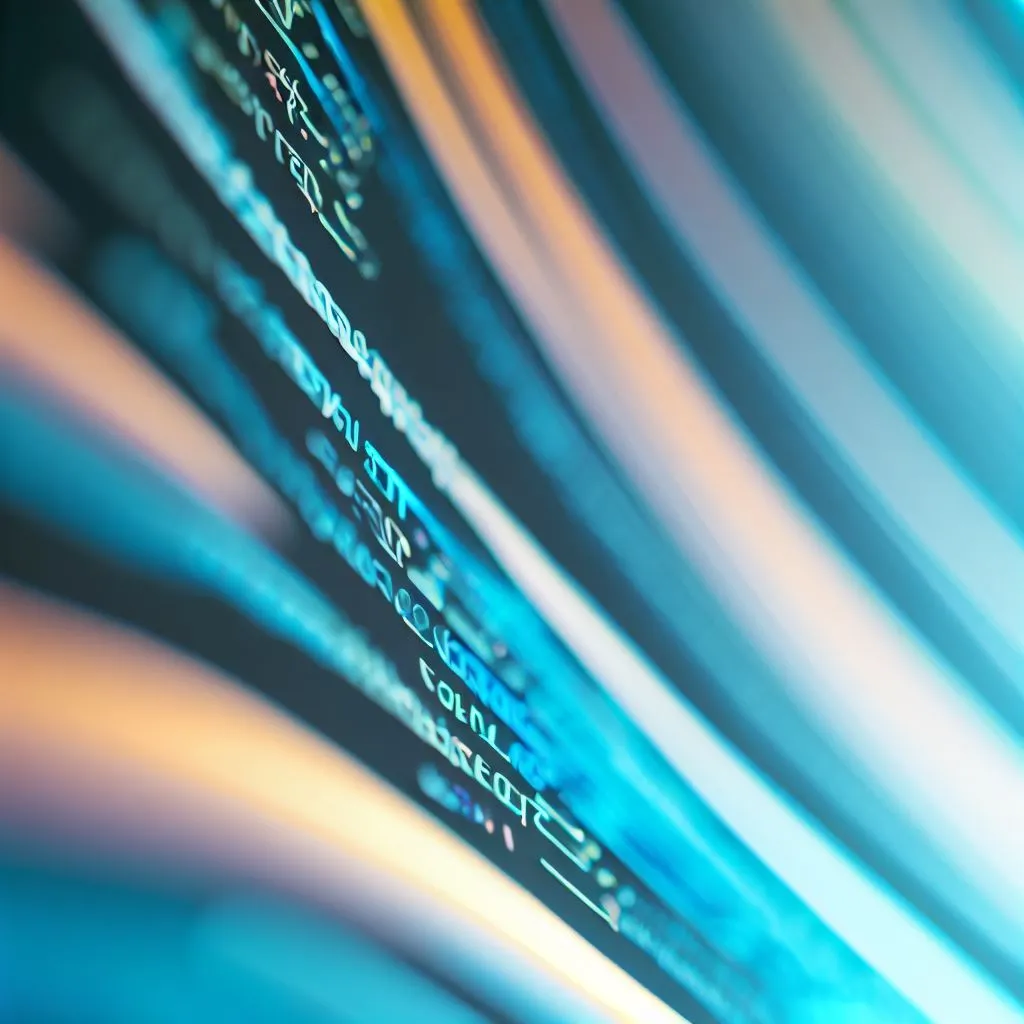
Since Microsoft introduced Modern Pages to Office 365 and SharePoint, it is really easy to create beautiful sites and pages without requiring any design experience.
If you need to customize the look and feel of modern pages, you can use custom tenant branding, custom site designs, and modern site themes without incurring the wrath of the SharePoint gods.
If you want to go even further, you can use SharePoint Framework Extensions and page placeholders to customize well-known areas of modern pages. Right now, those well-known locations are limited to the top and bottom of the page, but I suspect that in a few weeks, we’ll find out that there are more placeholder locations coming.
But what happens when your company has a very strict branding guideline that requires very specific changes to every page? When your customization needs go beyond what’s supported in themes? When you need to tweak outside of those well-known locations?
Or, what if you’re building a student portal on Office 365 and you need to inject a custom font in a page that is specifically designed to help users with dyslexia?
That’s when I would use a custom CSS.
Before you go nuts and start customizing SharePoint pages with crazy CSS customizations, we need to set one thing straight:
With SharePoint, you should always color within the lines. Don’t do anything that isn’t supported, ever. If you do, and you run into issues, you’re on your own.
With SharePoint, you should always color within the lines
Remember that Microsoft is constantly adding new features to SharePoint. The customizations you make with injecting custom CSS may stop working if the structure of pages change.
What’s worse, you could make changes to a page that prevents new features from appearing on your tenant because you’re inadvertently hiding elements that are needed for new features.
With custom CSS (and a CSS zen master), you can pretty much do anything you want. The question you should ask yourself is not whether you can do it, but whether it is the right thing to do.
It is very easy. In fact, I’m probably spending more time explaining how to do it than it took me to write the code for this. If you don’t care about how it works, feel free to download the source and install it.
Using SharePoint Framework Extensions, you can write code that you can attach to any Site, Web, or Lists. You can control the scope by how you register your extensions in your SharePoint tenant.
With an extension, you can insert tags in the HTML Head element.
I know what you’re thinking: we can just insert a STYLE block at in the HEAD element and insert your own CSS. Sure, but what happens when you need to change your CSS? Re-build and re-deploy your extension? Nah!
Instead, how about inserting a LINK tag and point to a custom CSS that’s located in a shared location? That way, you can modify the custom CSS in one place.
You can even have more than one custom CSS and use your extension properties to specify the URL to your custom CSS. In fact, you can add more than one extension on a site to combine multiple custom CSS together to suit your needs.
You too can design a beautiful SharePoint site that looks like this:
I’m really a better designer than this. I just wanted a screen shot that smacks you in the face with a bright red bar and a custom round site icon. It hurts my eyes.
Start by creating your own custom CSS (something better than I did, please). For example, the above look was achieved with the following CSS:
.ms-compositeHeader { background-color: red; } .ms-siteLogoContainerOuter { border-radius: 50%; border-width: 3px; } .ms-siteLogo-actual { border-radius: 50%; }
Save your custom CSS to a shared location on your SharePoint tenant. For example, you could save it in the Styles Library of your root site collection. You could also add it to your own Office 365 CDN. Make note of the URL to your CSS for later. For example, if you saved your custom CSS as contoso.css in the Styles Library of your tenant contoso.sharepoint.com, your CSS URL will be:
https://contoso.sharepoint.com/Style%20Library/contoso.css
which can be simplified to:
/Style%20Library/custom.css
export interface IInjectCssApplicationCustomizerProperties {
cssurl: string;
}
@override
public onInit(): Promise<void> {
Log.info(LOG_SOURCE, `Initialized ${strings.Title}`);
const cssUrl: string = this.properties.cssurl;
if (cssUrl) {
// inject the style sheet
const head: any = document.getElementsByTagName("head")[0] || document.documentElement;
let customStyle: HTMLLinkElement = document.createElement("link");
customStyle.href = cssUrl;
customStyle.rel = "stylesheet";
customStyle.type = "text/css";
head.insertAdjacentElement("beforeEnd", customStyle);
}
return Promise.resolve();
}
{
"$schema": "https://dev.office.com/json-schemas/core-build/serve.schema.json",
"port": 4321,
"https": true,
"serveConfigurations": {
"default": {
"pageUrl": "https://contoso.sharepoint.com/SitePages/Home.aspx",
"customActions": {
"fcea9230-7f22-45b7-815c-081a49474611": {
"location": "ClientSideExtension.ApplicationCustomizer",
"properties": {
"cssurl": "/Style%20Library/custom.css"
}
}
}
},
"injectCss": {
"pageUrl": "https://contoso.sharepoint.com/SitePages/Home.aspx",
"customActions": {
"fcea9230-7f22-45b7-815c-081a49474611": {
"location": "ClientSideExtension.ApplicationCustomizer",
"properties": {
"cssurl": "/Style%20Library/custom.css"
}
}
}
}
}
}
You can now tweak your custom CSS to suit your needs, continuing to hit refresh until you’re happy with the results.
When ready to deploy, you need to bundle your solution, upload it to the app catalog, and enable the extension on every site you want to customize.
To make things easy, you can add an elements.xml file in your SharePoint folder and pre-configure your custom CSS URL. Here’s how:
In your solution’s sharepoint/assets folder, create a new file called elements.xml. If you don’t have a sharepoint folder or assets sub-folder, create them.
Paste the code below in your elements**.xml**:
<?xml version="1.0" encoding="utf-8"?>
If you selected to automatically deploy to all site collections when building the extension, you’re done. If not, you’ll need to go to every site and add the extension by using the Site Contents and Add an App links.
You can easily inject custom CSS in every modern page of your SharePoint tenant by using an SPFx extension, but be careful. With great CSS power comes great SharePoint responsibility.
You can get the code for this extension at https://github.com/hugoabernier/react-application-injectcss
I’d love to see what you’re doing with your custom CSS. Let me know in the comments what you have done, and — if you’re interested — share the CSS.
I hope this helps?